Member Banner
Allows your customers to check their AIR MILES account balances and details right from your mobile application or website.
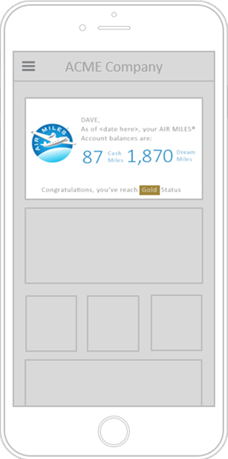
Generating an Authorization Code
Generate an Authorization Code by redirecting your customer to our universal login page.
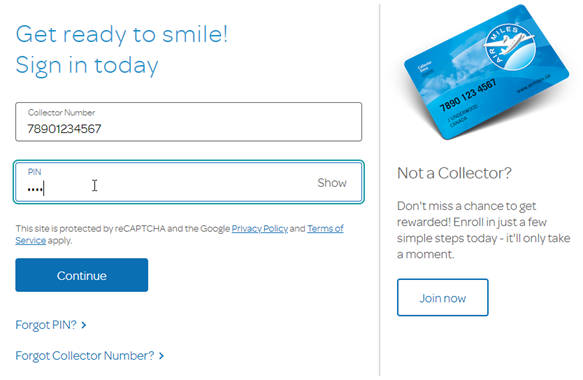
(Fig. 1) AIR MILES universal log in page
Sample Authorization Request
<a href="https://oauth-cert.airmiles.ca/authorize?
client_id=knPJldKv8ygPn56gZhdFEIZV27QevLkx&
response_type=code&
connection=member-pin-idp-recaptcha&
redirect_uri=https://your_redirect_uri/callback&
scope=memberbanner%20offline_access&
audience=https://members.loyalty.com">
Sign In
</a>
Parameter | Description |
---|---|
client_id |
To be provided by AIR MILES. |
response_type |
Do not change, keep as code . |
connection |
Do not change, keep as member-pin-idp-recaptcha . |
redirect_uri |
The URL which Auth0 will redirect the browser to, and send the authorization code.
Note The address must be a valid callback URI, and needs to be communicated to AIR MILES at the same time yourclient_id is being setup.
|
scope |
Do not change, keep as memberbanner .
Note Theoffline_access scope enables the use of Refresh Tokens and is optional
|
audience |
Do not change, keep as https://members.loyalty.com . |
Sample Authorization Response
Once your customer authenticates themselves, a response will be sent to the redirect URI that includes an Authorization Code.
HTTP/1.1 302 Found
Location: https://your_redirect_uri/callback?code=6hgDftTGH656HEDLdfOPA43nmsR5GuqG
Generating an Access Token
An Access Token is required to communicate with the Member Banner API. To generate an Access Token (aka JSON Web Token), make an API request like the one shown below.
WARNING
The Access Token request must originate from your backend, otherwise it will be rejected by Auth0. If for example the request originates from a Web browser, it will be declined, as this means yourclient_secret
has been exposed to the public.
Sample Token Request
curl --request POST \
--url 'https://oauth-cert.airmiles.ca/oauth/token' \
--data-urlencode 'grant_type=authorization_code' \
--data-urlencode 'client_id=knPJldKv8ygPn56gZhdFEIZV27QevLkx' \
--data-urlencode 'client_secret=lfgdFGDF456fFDFV4FdDFffdl7h8J43s' \
--data-urlencode 'code=6hgDftTGH656HEDLdfOPA43nmsR5GuqG' \
--data-urlencode 'redirect_uri=https://your_redirect_uri/callback'
Parameter | Description |
---|---|
grant_type |
Do not change, keep as authorization_code . |
client_id |
The client_id used in the previous step. |
client_secret |
To be provided by AIR MILES. |
code |
The Authorization Code (i.e., code ) returned in the last step. |
redirect_uri |
The redirect_uri used in the previous step. |
Sample Token Response
If all goes well, you’ll receive a (HTTP 200) JSON response with a payload containing an access_token
, refresh_token
, id_token
, and token_type
.
{
"access_token": "eyJz93a409sSwFG8kkIL2qsGHk4laUWw",
"refresh_token": "GE5g5HJdbYb47HN358polTHdf3tHf3ra",
"id_token": "eyKMD32ldkgfkDYKL4h6hf33edHGSDKk",
"token_type": "Bearer"
}
Notes on Refresh Tokens
- The
refresh_token
will only be present in the response if you included theoffline_access
scope. - Refresh Tokens must be stored securely since they allow a user to remain authenticated essentially forever.
JSON Web Token (JWT) structure
The JWT consists of three concatenated Base64url-encoded strings, separated by dots “.
”.
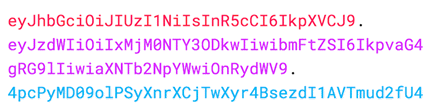
(Fig. 2) JWT Example
String | Description |
---|---|
Header | Contains metadata about the type of token and the cryptographic algorithms used to secure its contents. |
Payload | Contains verifiable security statements, such as the Collector ID and the allowed scopes. |
Signature | Used to validate that the token is trustworthy and has not been tampered with. |
Calling the Member Banner API
Finally, to display the Collector’s account details, make an API request using the Access Token received in the previous step.
Sample API Request
curl -X GET \
`https://cert.airmilesapis.ca/member-profile/banner` \
-H 'Authorization: Bearer eyJz93a409sSwFG8kkIL2qsGHk4laUWw' \
Tip
Make sure to include the wordBearer
before the token, as shown in the example above.
Sample API Response
If everything went as expected you should receive a JSON response that includes the Collector’s account details, as shown in the example below.
{
"cardNumber": "84151103449",
"memberId": "fbd651ea-af99-3a71-b0db-83d4feae087f",
"firstName": "JIMMY",
"tier": "Blue",
"cashBalance": 10901,
"dreamBalance": 849
}
Parameter | Description |
---|---|
cardNumber |
The Collector’s AIR MILES card number. |
memberId |
The Collector’s ID number. |
firstName |
The Collector’s first name (given name). |
tier |
The Collector’s account level (Blue, Gold, or Onyx). |
cashBalance |
The Collector’s balance of AIR MILES Cash Miles. |
dreamBalance |
The Collector’s balance of AIR MILES Dream Miles. |
Using a Refresh Token
Typically, a user needs a new access token when gaining access to a resource for the first time, or after the previous access token granted to them expires. A refresh token is a special kind of token used to obtain a renewed access token. You can request new access tokens until the refresh token is blacklisted. Applications must store refresh tokens securely because they essentially allow a user to remain authenticated forever.
Note
To successfully obtain a refresh token, you must first have included theoffline_access
scope when you initiated your authentication request through the ../authorize
endpoint, as shown in Step 1.
To exchange the Refresh Token you received during authorization for a new Access Token, make a POST
request to https://oauth-cert.airmiles.ca/oauth/token
, using grant_type=refresh_token
as seen in the example below.
Important
You should only ask for a new token if the access token has expired or you want to refresh the claims contained in the ID token. For example, it’s bad practice to call the endpoint to get a new access token every time you call an API. There are rate limits in Auth0 that will throttle the number of requests to this endpoint that can be executed using the same token from the same IP.Sample ‘Refresh Token’ Request
curl --request POST \
--url 'https://oauth-cert.airmiles.ca/oauth/token' \
--header 'content-type: application/x-www-form-urlencoded' \
--data grant_type=refresh_token \
--data-urlencode 'client_id=knPJldKv8ygPn56gZhdFEIZV27QevLkx' \
--data-urlencode client_secret=lfgdFGDF456fFDFV4FdDFffdl7h8J43s \
--data refresh_token=YOUR_REFRESH_TOKEN
Parameter | Description |
---|---|
grant_type |
Do not change, keep as refresh_token . |
client_id |
To be provided by AIR MILES. |
client_secret |
To be provided by AIR MILES. |
refresh_token |
The Refresh Token to use. |
Note
The scopes requested in the initial request are included by default in the Refresh Token.Sample ‘Refresh Token’ Response
If all goes well, you’ll receive an HTTP 200
response with a payload containing a new access_token
, its lifetime in seconds (expires_in
), granted scope
values, and token_type
. If the scope of the initial token included openid
, then the response will also include a new id_token
:
The response will include a new Access Token, its type, its lifetime (in seconds), and the granted scopes. The memberbanner
scope should also be included in the response as well.
{
"access_token": "eyJz93a409sSwFG8kkIL2qsGHk4laUWw",
"scope": "memberbanner offline_access",
"id_token": "eyKMD32ldkgfkDYKL4h6hf33edHGSDKk",
"token_type": "Bearer"
}
AIR MILES Brand Guidelines
Our Brand Guidelines site is currently offline for ongoing updates and maintenance. In the meantime, please reach out to our ‘Brand Approvals’ team in the ‘Rewards & Marketing’ department, to request more information on website and application integration.
Contact information
-
- Email for Brand Approvals
- brandapprovals@loyalty.com